Mon May 08 2023
Array Iteration in JavaScript: Using reduce, reduceRight, every, and some
JavaScript provides a rich set of methods to iterate and manipulate arrays, making it easier for developers to work with collections of data. Among these methods, reduce, reduceRight, every, and some are particularly useful for performing advanced operations on arrays. Each has a unique purpose and use case, and understanding their behavior can help streamline code and improve its readability. In this article, I’ll show you how each of these array methods works and when you might use them in your JavaScript applications.
1. reduce() Method
The reduce method is used to iterate over an array and accumulate a single output value based on a function you provide. This method is highly flexible and can be used for various purposes, such as summing numbers, transforming data, and even flattening arrays.
const sum = num.reduce((total, value, index, array) => total+value);
document.getElementById('reduceData').innerHTML = 'Sum From Left Side: ' + sum;
2. reduceRight() Method
The reduceRight method is similar to reduce, but it iterates over the array elements from right to left. This can be useful when you need to accumulate values in reverse order. While reduce is more commonly used, reduceRight is handy when you need to work from the end of an array.
const sum = num.reduceRight((total, value, index, array) => total + value);
document.getElementById('rRightData').innerHTML = 'Sum From Left Side: ' + sum;
3. every() Method
The every method checks if all elements in an array meet a certain condition. It returns true if every element in the array passes the test function you provide; otherwise, it returns false. The every method is useful for validation checks, such as ensuring that all items meet specific criteria.
const allOver = num.every((value) => value > 50);
document.getElementById('everyData').innerHTML = allOver;
4. some() Method
The some method checks if at least one element in an array meets a certain condition. It returns true if any element passes the test function; otherwise, it returns false. The some method is beneficial when you only need one element in the array to match a condition, such as checking for the presence of specific attributes or flags.
const isTrue = num.some((value) => value < 80);
document.getElementById('someData').innerHTML = isTrue;
Conclusion
By understanding these methods, you can perform powerful and efficient data manipulations in JavaScript. Each of them provides cleaner syntax and more expressive code for common tasks that otherwise would require loops and extra variables. Use these methods effectively to write more concise, readable, and functional code in JavaScript.

File Name: js-array-iteration2.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Array Iteration in JavaScript: Part 2</title>
<style>
section {
display: flex;
gap: 10;
justify-content: space-between;
}
section div.col {
width: calc(100% / 4);
}
section div.col > div {
margin: 10px 0;
}
button { margin-bottom: 10px; }
</style>
<script>
let num = [25, 45, 81, 64, 10, 46, 89, 49, 22, 40, 78, 62];
const getReduce = () => {
const sum = num.reduce((total, value, index, array) => total+value);
document.getElementById('reduceData').innerHTML = 'Sum From Left Side: ' + sum;
}
const getReduceRight = () => {
const sum = num.reduceRight((total, value, index, array) => total + value);
document.getElementById('rRightData').innerHTML = 'Sum From Left Side: ' + sum;
}
const getEvery = () => {
const allOver = num.every((value) => value > 50);
document.getElementById('everyData').innerHTML = allOver;
}
const getSome = () => {
const isTrue = num.some((value) => value < 80);
document.getElementById('someData').innerHTML = isTrue;
}
</script>
</head>
<body>
<section>
<div class="col">
<button type="button" onclick="getReduce()">Reduce Iteration</button>
<div id="reduceData"></div>
</div>
<div class="col">
<button type="button" onclick="getReduceRight()">Reduce Right Iteration</button>
<div id="rRightData"></div>
</div>
<div class="col">
<button type="button" onclick="getEvery()">Every Iteration</button>
<div id="everyData"></div>
</div>
<div class="col">
<button type="button" onclick="getSome()">Some Iteration</button>
<div id="someData"></div>
</div>
</section>
</body>
</html>
Result Screenshot(s)
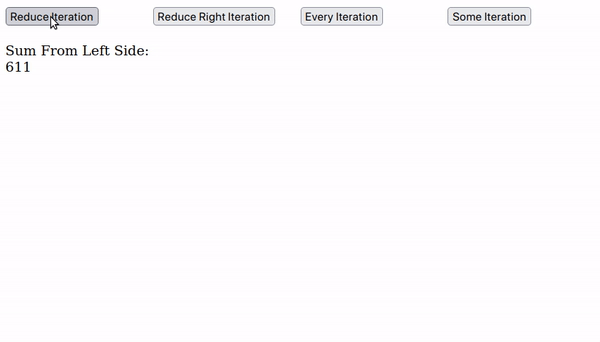