Fri Mar 10 2023
Function Syntax
JavaScript71 views
File Name: javascript-function-syntax.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Function Syntax</title>
<script>
/* Tradition Function */
function sum1(x, y) {
return x+y;
}
/* Anonymous Function Assigned to a Variable */
const sum2 = function (x, y) {
return x + y;
}
/* Arrow Function */
const sum3 = (x, y) => {
return x + y;
}
function calculator() {
document.getElementById('fun1').innerHTML = sum1(5, 6);
document.getElementById('fun2').innerHTML = sum2(6, 7);
document.getElementById('fun3').innerHTML = sum3(7, 8);
}
</script>
</head>
<body>
<button onclick="calculator()">Calculate</button>
<h1>Traditional Function: <span id="fun1"></span></h1>
<h1>Anonymous Function: <span id="fun2"></span></h1>
<h1>Arrow Function: <span id="fun3"></span></h1>
</body>
</html>
Result Screenshot(s)
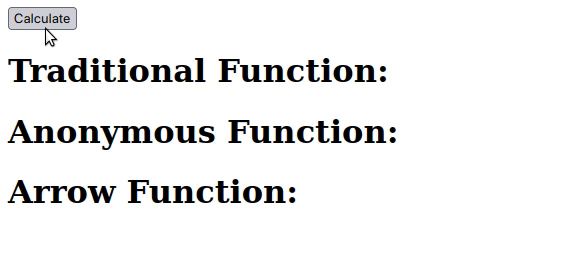
Author:Geekboots