Sat May 06 2023
Array Iteration Part 1
JavaScript91 views

File Name: js-array-iteration.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Array Iteration in JavaScript</title>
<style>
section {
display: flex;
gap: 10;
justify-content: space-between;
}
section div.col {
width: calc(100% / 4);
}
section div.col > div {
margin: 10px 0;
}
button { margin-bottom: 10px; }
</style>
<script>
let num = [25, 45, 81, 64, 10, 46, 89, 49, 22, 40, 78, 62];
const getForEach = () => {
num.forEach((value, index) => {
document.getElementById('forEData').innerHTML += index + ' - ' + value +'<br />';
})
}
const getMap = () => {
num.map((value, index) => {
document.getElementById('mapData').innerHTML += index + ' - ' + value +'<br />';
})
}
const getFlatMap = () => {
const newArray = num.flatMap((value) => value + 2);
document.getElementById('flatMapData').innerHTML = newArray;
}
const getFilter = () => {
const newArray = num.filter((value) => value > 40);
document.getElementById('filterData').innerHTML = newArray;
}
</script>
</head>
<body>
<section>
<div class="col">
<button type="button" onclick="getForEach()">ForEach Iteration</button>
<div id="forEData"></div>
</div>
<div class="col">
<button type="button" onclick="getMap()">Map Iteration</button>
<div id="mapData"></div>
</div>
<div class="col">
<button type="button" onclick="getFlatMap()">Flat Map Iteration</button>
<div id="flatMapData"></div>
</div>
<div class="col">
<button type="button" onclick="getFilter()">Filter Iteration</button>
<div id="filterData"></div>
</div>
</section>
</body>
</html>
Result Screenshot(s)
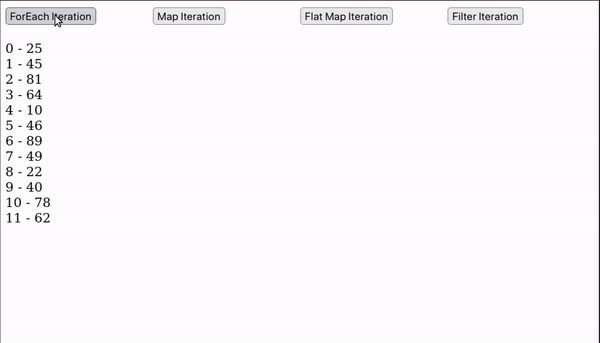
Author:Geekboots