Fri May 06 2022
How to Create Text Loading Animation in HTML and CSS
Text loading animations are commonly used in modern websites to give users visual feedback while the content is being loaded. In this tutorial, we’ll follow the steps to create a simple, yet effective, text loading animation with text filling effect using only HTML and CSS. No JavaScript is needed for this, making it lightweight and easy to implement.
Step 1: Basic HTML Structure
<section>
<h1 data-text="Geekboots...">Geekboots...</h1>
</section>
Step 2: Add CSS for Styling and Animation
Now, we'll use CSS to style the text and create the loading animation. In the following CSS, we'll use @keyframes to animate the text. Also use the before pseudo element and text-stroke to show text with stroke color.
h1 {
position: relative;
font-size: 80px;
font-weight: 400;
color: #4d4d4d;
-webkit-text-stroke: 2px #666666;
}
h1::before {
position: absolute;
content: attr(data-text);
top: 0;
left: 0;
width: 100%;
height: 100%;
color: #05a9f3;
-webkit-text-stroke: 0px #05a9f3;
overflow: hidden;
animation: loading 6s ease-out forwards;
}
@keyframes loading {
0% { width: 0; }
20% { width: 45%; }
80% { width: 75%; }
90% { width: 85%; }
100% { width: 100%; }
}
Conclusion
In this tutorial, we created a simple and effective text loading animation using just HTML and CSS. The animation is achieved through the clever use of CSS @keyframes, before pseudo, and text stroke properties, providing an engaging visual feedback element for your users while content is loading. You can further customize this animation to match your website’s design, alter the timing, or even combine it with more complex animations using JavaScript or other CSS properties.
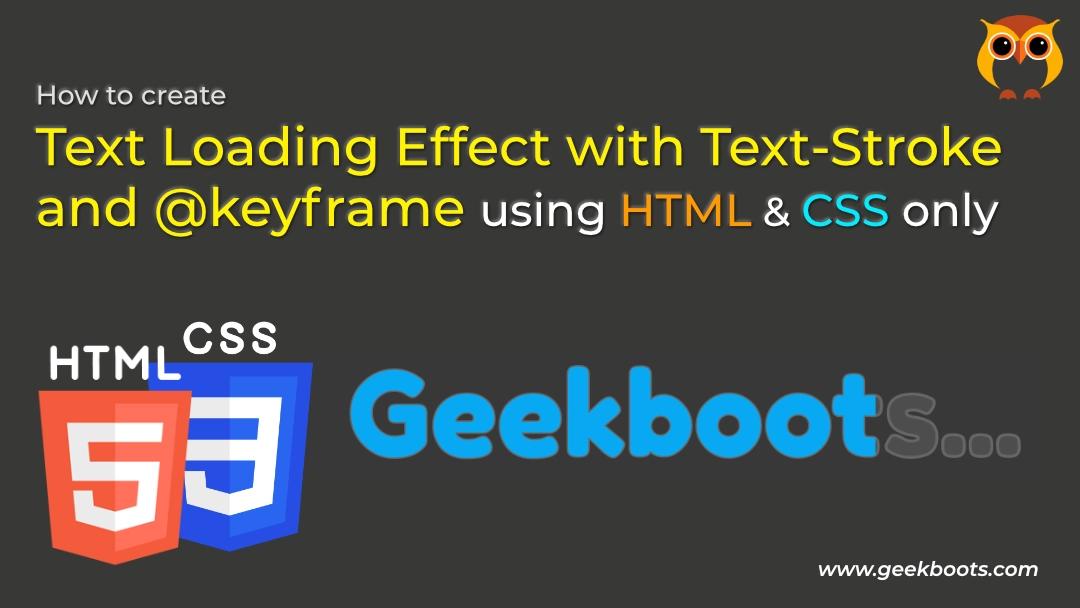
File Name: Text-Loading-Effect.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Loading Text</title>
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Fredoka+One&display=swap" rel="stylesheet">
<style>
* {
margin: 0;
padding: 0;
font-family: 'Fredoka One', cursive;
}
body {
background-color: #383838;
}
section {
width: 100%;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
}
h1 {
position: relative;
font-size: 80px;
font-weight: 400;
color: #4d4d4d;
-webkit-text-stroke: 2px #666666;
}
h1::before {
position: absolute;
content: attr(data-text);
top: 0;
left: 0;
width: 100%;
height: 100%;
color: #05a9f3;
-webkit-text-stroke: 0px #05a9f3;
overflow: hidden;
animation: loading 6s ease-out forwards;
}
@keyframes loading {
0% { width: 0; }
20% { width: 45%; }
80% { width: 75%; }
90% { width: 85%; }
100% { width: 100%; }
}
</style>
</head>
<body>
<section>
<h1 data-text="Geekboots...">Geekboots...</h1>
</section>
</body>
</html>
Result Screenshot(s)
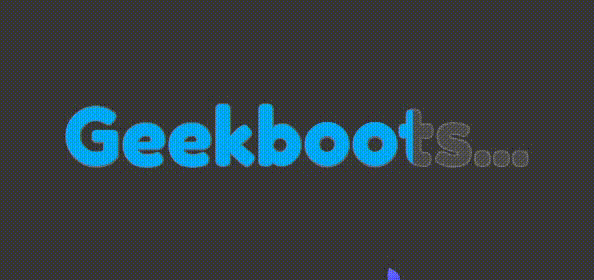